DXR is now supported on GTX cards, RTX cards are 2 to 3 times faster ..
https://wccftech.com/nvidia-geforce-game-ready-driver-geforce-gtx-ray-tracing-rtx-features-enabled/
Time for some Titan XP SLI benchmarking...
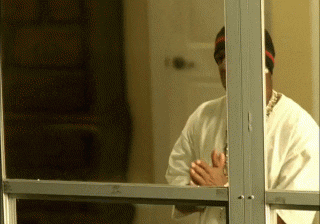
Follow along with the video below to see how to install our site as a web app on your home screen.
Note: This feature may not be available in some browsers.
DXR is now supported on GTX cards, RTX cards are 2 to 3 times faster ..
https://wccftech.com/nvidia-geforce-game-ready-driver-geforce-gtx-ray-tracing-rtx-features-enabled/
Well if rumours are to be believed.Do you think AMD will follow soon ?
Yes.Do you think AMD will follow soon ?
Nvidia says that its latest Game Ready driver allows tens of million of GeForce GTX owners to test drive real-time ray tracing in games that support DXR. And while that’s true, you have to realize that even best-case performance (Titan Xp) in the least-intensive workload we tested (Battlefield V) is cut in half on Pascal-based cards.
If you go for an uniform tree, you have hardly any choice but to record triangles as leaf nodes to more than one intermediate tree-node. It's a trade-off between space saved on the leaf nodes and complexity of traversal / near misses. Loose octtree and geometry on intermediate nodes complicates traversal significantly. Especially loose octtree is going to amplify necessary memory transactions.If you link each triangle to only one node, and take the advantage to store no node positions and bounding volumes (which makes octree so attractive), then you have to assume a loose octree, with each bounding box having 8 times the volume than the grid cell of the node.
That's not something I'm worried about at all. You can compress it down to 1bit per cell for encoding empty spaces, and then index by hardwired pop-count into linear storage of occupied cells only. Each child node should be usually unique within the list of children, so no further compression applies. Should work ideally for a 8x8x4 (the 4 rotating axis by level) node size if you wanted to exactly fit a 32 byte cache line for the bitset, followed by packed indices. If you like, you can also encode metadata for the next child within the packed field, in order to cut on waste on the second memory access.New If you look at the traversal gains to be had simply by making the boxes of unused space larger in AABBs I'm not sure straight octrees are a good idea.
Nvidia's optimization guidelines mentioned something about degeneration of the acceleration structures, so you were urged to rebuild occasionally. In hindsight, that gave it away. Not many options for acceleration structures which can degenerate on an update to begin with, and even less which would be suitable for GPU accelerated updates. Only part we can't deduce for now is the precise data layout for each node, but we can safely say it contains a bounding box (unclear if all 3 or only 2 ordinates are encoded per level?) and 2-4 child indices, plus some metadata to distinguish tree nodes from leaf nodes. Metadata may be encoded in index, rather than inside the node. Possible layout may be 6x4byte for BB, plus 2x4byte for child indices, with 1-2 bit per index reserved for metadata encoding child properties.We don't know how their tree looks like. [...] But NV is unknown?
How would you do this step efficiently? Sounds very expensive. Assuming we already know which leaf nodes are necessary, two steps remain:If you go for an uniform tree, you have hardly any choice but to record triangles as leaf nodes to more than one intermediate tree-node.
Do any GPU/cuda ray tracing engines *not* use BVH? Pretty much every piece of literature and source I've seen in the GPU-RT space seems to prefer BVH.
https://www.overclock3d.net/news/so...acing_at_gdc_-_amd_and_nvidia_will_be_there/1To date, only one retail game has delivered raytracing support through the Vulkan API, Wolfenstein Youngblood. If you want to include more games in this list, you'll need to include Vulkan-based projects like Quake II RTX and other non-retail releases.
As it stands, the Vulkan API lacks support for raytracing. Yes, Nvidia has released RTX specific extensions for Vulkan raytracing, but this support is hardware exclusive and lacks the multi-platform/multi-vendor raytracing support that Microsoft's DXR (DirectX Raytracing) delivers.
Sadly, we do not know what form Khronos' ray tracing implementation will take. Still, given Khronos' recent moves with Vulkan 1.2, we guess that Vulkan will closely align with Microsoft and its DXR implementation. Vulkan 1.2 already supports HLSL (DirectX's Shading Language) with support for up to Shader Model 6.2. Support for Shader Model 6.3 will bring with it support for DXR HLSL code, and this code should be usable with Vulkan's planned raytracing implementation.